Game Example (Use Case)¶
This document provides a step-by-step guide to create a new game and several tasks within that game, utilizing different gamification strategies through our API.
Retrieve All Strategies¶
To begin, it’s necessary to know which strategy you will apply to the game you’re creating. For this purpose, you can list all the strategies using the following endpoint.
Endpoint: GET /api/v1/strategies
Example of response:
[
{
"id": "socio_bee",
"name": "SOCIO_BEE",
"description": "A more advanced gamification strategy with additional points and penalties.",
"version": "0.0.2",
"variables": {
"variable_basic_points": 1,
"variable_bonus_points": 10,
"variable_individual_over_global_points": 3,
"variable_peak_performer_bonus_points": 15,
"variable_global_advantage_adjustment_points": 7,
"variable_individual_adjustment_points": 8
}
},
{
"id": "default",
"name": "EnhancedGamificationStrategy",
"description": "A more advanced gamification strategy with additional points and penalties.",
"version": "0.0.2",
"variables": {
"variable_basic_points": 1,
"variable_bonus_points": 10,
"variable_individual_over_global_points": 3,
"variable_peak_performer_bonus_points": 15,
"variable_global_advantage_adjustment_points": 7,
"variable_individual_adjustment_points": 8
}
}
]
Graphical representation:
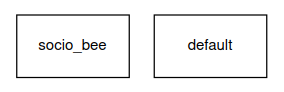
Create a Game¶
To start, you need to create a game. A game serves as a container for one or more tasks that you can assign to users.
Endpoint: POST /api/v1/games
Request Body:
{
"externalGameId": "example_game",
"platform": "example_platform",
"strategyId": "socio_bee",
"params": [
{
"key": "variable_basic_points",
"value": 5
}
]
}
Example of response:
{
"gameId": "9b22b0f1-ecbf-442f-9574-222ad9b9e262",
"created_at": "2024-04-08T09:49:24.269447+00:00",
"updated_at": "2024-04-08T09:49:24.269447+00:00",
"externalGameId": "example_game",
"strategyId": "socio_bee",
"platform": "example_platform",
"params": [
{
"key": "variable_basic_points",
"value": "5",
"id": "f4ba61aa-b35f-4d89-9075-94d4a0453180"
}
],
"message": "Game with gameId: 9b22b0f1-ecbf-442f-9574-222ad9b9e262 created successfully"
}
externalGameId: This is an identifier external to the GAME system, used to identify the game in the future if necessary. This ID is for informational purposes only and will not be used in the system’s internal logic.
variable_basic_points is set to a value of 5. This value can be modified in the future for this game.
This body of the request creates a game with the “socio_bee” strategy, where the basic points variable is initially set to 5. This setup allows for a flexible and dynamic approach to game creation, enabling the modification of game parameters as needed.
IMPORTANT: The game ID (gameId) is generated by the system and is unique for each game. This ID is used to reference the game in the future, so it is important to store it for future reference.
Graphical representation:
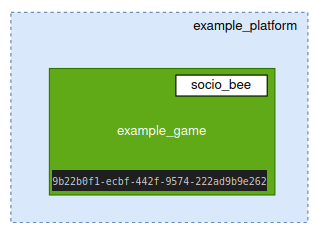
Create task with game strategy¶
After creating a game, the next step is to add tasks to it. Tasks represent specific activities or actions that users are expected to complete within the game. A task can either inherit the game’s overall strategy or be configured with its specific parameters, even if it follows the inherited strategy.
Endpoint: POST /api/v1/games/{gameId}/tasks
Replace {gameId}
with the actual ID of the game to which you want to add the task.
Request Body:
{
"externalTaskId": "example_task",
"params": [
{
"key": "variable_basic_points",
"value": 10
}
]
}
Example of response:
{
"message": "Task created successfully with externalTaskId: example_task for gameId: 9b22b0f1-ecbf-442f-9574-222ad9b9e262 ",
"externalTaskId": "example_task",
"externalGameId": "example_game",
"gameParams": [
{
"key": "variable_basic_points",
"value": "5"
}
],
"taskParams": [
{
"key": "variable_basic_points",
"value": "10"
}
],
"strategy": {
"id": "default",
"name": "EnhancedGamificationStrategy",
"description": "A more advanced gamification strategy with additional points and penalties.",
"version": "0.0.2",
"variables": {
"variable_basic_points": 10,
"variable_bonus_points": 10,
"variable_individual_over_global_points": 3,
"variable_peak_performer_bonus_points": 15,
"variable_global_advantage_adjustment_points": 7,
"variable_individual_adjustment_points": 8
}
}
}
externalTaskId: A unique identifier for the task, external to the GAME system. This ID is used to identify the task in the future if necessary.
This request demonstrates how to set a specific value for “variable_basic_points” for a particular task. By doing so, this task is configured to award 10 basic points to users upon completion. It’s important to note:
Inheriting the Game Strategy: By not specifying a “strategyId” in the request, the task inherits the strategy of its parent game. If the game’s strategy includes a default value for “variable_basic_points”, this specific task overrides that default with its own value of 10.
Task-Specific Parameters: The “params” section allows for setting task-specific parameters. In this case, “variable_basic_points” is set to 10, demonstrating that tasks can have unique configurations independent of the game’s overall settings or other tasks.
This flexibility in task creation ensures that while tasks can align with the broad gamification strategy of their parent game, they can also be individually tailored to meet specific goals or requirements. This approach allows for a rich and varied user experience within the same game framework.
Graphical representation:
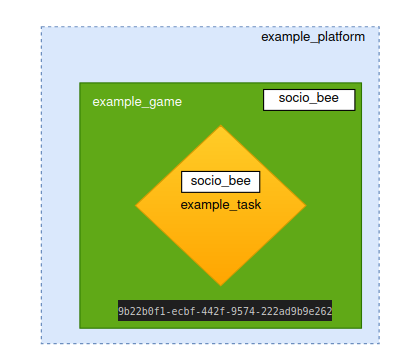
Create task with custom strategy¶
After setting up your game, you might want to add tasks that, while inheriting the game’s general strategy, use a specific, independent strategy for more tailored gamification approaches. This method allows for custom task-level strategies that may vary from the game’s default strategy but still adhere to the overarching gamification framework.
Endpoint: POST /api/v1/games/{gameId}/tasks
Ensure to replace {gameId}
with the actual ID of the game to which you’re adding the task.
Request Body:
{
"externalTaskId": "example_task_with_own_strategy",
"strategyId": "custom_strategy",
"params": [
{
"key": "variable_basic_points",
"value": 15
}
]
}
Example of response:
{
"message": "Task created successfully with externalTaskId: example_task_with_own_strategy for gameId: example_game_id",
"externalTaskId": "example_task_with_own_strategy",
"externalGameId": "example_game",
"gameParams": [
{
"key": "variable_basic_points",
"value": "5"
}
],
"taskParams": [
{
"key": "variable_basic_points",
"value": "15"
}
],
"strategy": {
"id": "custom_strategy",
"name": "Custom Strategy Name",
"description": "A custom strategy designed for specific task engagement.",
"version": "1.0.0",
"variables": {
"variable_basic_points": 15,
"variable_bonus_points": 20,
"variable_individual_over_global_points": 5,
"variable_peak_performer_bonus_points": 25,
"variable_global_advantage_adjustment_points": 10,
"variable_individual_adjustment_points": 12
}
}
}
externalTaskId: A unique identifier for the task, used to identify the task in future interactions.
This request specifies a custom strategy for the task by using the “strategyId” field. Even though a specific strategy is applied, it’s crucial to understand:
Inheritance of Variables: Regardless of the defined strategy, variables from the game’s default strategy can still be inherited unless explicitly overridden in the task’s parameters. In this example, “variable_basic_points” is set to 15, explicitly overriding the game’s default or any previously inherited value for this variable.
Strategy Independence: Specifying a “strategyId” allows this task to implement a strategy that is different from the game’s default. This enables more nuanced gamification mechanics tailored to the task’s specific goals or behaviors, providing a diverse user experience within the same game environment.
This method of task creation enhances the flexibility and depth of the gamification system, allowing for both broad strategy alignment and specific task-level customizations.
Graphical representation:
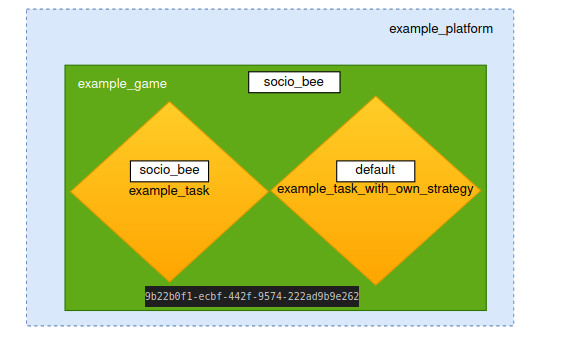
Assign Points to a User in a Game¶
After creating tasks within your game, assigning points to users based on their task completion is a crucial next step. Our system offers the flexibility to assign points to users even if they have not been previously registered in the system. If a user does not exist at the time points are assigned, the system will automatically create a profile for them. This ensures that users can have a presence in one or more games without needing explicit prior creation.
Endpoint: POST /api/v1/games/{gameId}/tasks/{taskId}/points/assign
To assign points to user “user_1” for completing the task “example_task” in the game with gameId
“9b22b0f1-ecbf-442f-9574-222ad9b9e262”, follow the steps below. Remember to replace {gameId}
and {taskId}
with their actual values.
Request Body:
{
"externalUserId": "user_1",
"data": {}
}
Example of Response:
{
"points": 1,
"caseName": "BasicEngagement",
"isACreatedUser": true,
"gameId": "9b22b0f1-ecbf-442f-9574-222ad9b9e262",
"externalTaskId": "example_task",
"created_at": "2024-04-08 12:05:37.161918+00:00"
}
externalUserId: The identifier for the user receiving points. If “user_1” does not exist, the system will automatically create a new profile for them.
caseName: Represents the name of the condition within the strategy that was met to assign points. This field helps identify which conditions were fulfilled to award points under the game’s strategy.
isACreatedUser: This boolean indicates whether the user was created as part of this point assignment process (true if the user was not previously registered and was thus created, false otherwise).
This response confirms that points were successfully assigned, providing details about the transaction. It includes whether a new user profile was created, aligning with the system’s ability to dynamically integrate users into the game environment. This feature ensures a seamless and inclusive gamification strategy, accommodating dynamic user engagement across multiple games and tasks without the need for prior user setup or registration.
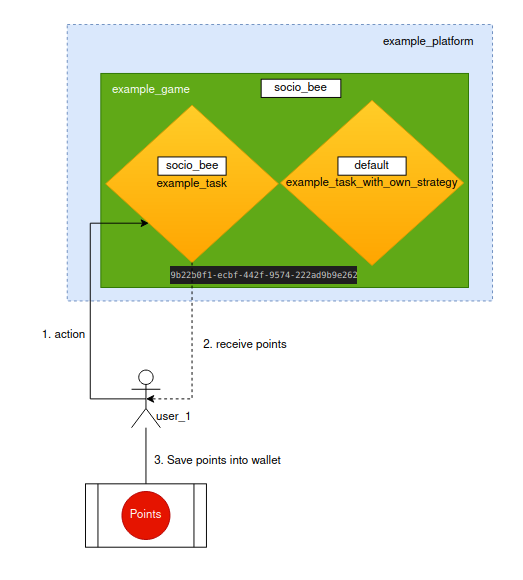